Data integrity refers back to the assurance that information stays correct, unaltered, and constant all through its lifecycle. In communication, it’s essential to make sure information integrity to safeguard in opposition to unintended alterations and malicious interventions throughout transmission.
The integrity of Digital Data is completed utilizing Hashing Algorithms. The crypto
module in Node gives numerous built-in vetted library capabilities to supply means to not solely confirm the integrity of information but additionally the authenticity of its origin.
This handbook goals to spotlight the inner workings of the capabilities within the crypto
library and offer you some insights into the inner workings of HMAC and Diffie-Hellman Key Exchange. This will aid you make knowledgeable selections about hash algorithms and key lengths relying on your online business necessities.
The main focus of this handbook is to emphasise the essential facet of information integrity somewhat than discussing the assorted encryption algorithms out there.
Encryption is used to guard data by changing it right into a safe format, which ensures its confidentiality. But information integrity is worried with guaranteeing that the info stays correct and unaltered.
You can even watch the related video right here:
Table of Contents
Prerequisites
- Node and Express: We’ll create a TypeScript pattern utility utilizing the Express framework. A primary understanding of the framework can be useful. You will want the Node Runtime Environment to execute the scripts.
- Postman Client: To make an API request and to check out the pattern utility, you have to a software to make HTTP Requests. You could use your net browser’s “Edit and Send” characteristic underneath the Networks tab, however since not all browsers permit this, it is best to make use of a software like Postman which gives a greater UI to watch responses.
The Alice-Bob Paradigm
Throughout this handbook you’ll come throughout quite a few sequence diagrams and mathematical proofs that use the Alice-Bob Paradigm.
The Alice-Bob paradigm is a standard conference in cryptography the place two generic entities, usually named Alice and Bob, are used for example numerous situations, protocols, or cryptographic rules.
These characters symbolize two events engaged in communication, with Alice sometimes representing the sender or initiator, and Bob representing the receiver or responder.
We usually introduce Eve as a 3rd social gathering, symbolizing an eavesdropper or potential attacker, including a component of safety danger and illustrating situations the place exterior entities would possibly try and intercept or manipulate the communication.
The pattern utility proven within the later sections fashions after this Alice-Bob Paradigm to make use of Boost Inc. and Account Aggregator (AA) because the events engaged in communication.
Message Detection Code (MDC)
When Alice must ship essential information to Bob over the web, the info adjustments arms, leaping between routers and servers, every step carrying the potential danger of unintended alterations.
If Eve manages to get their arms on Alice’s information, they could modify it. So the integrity of the info turns into questionable, emphasizing that its unique state could have been compromised throughout transmission.
Note that we’re speaking concerning the integrity and never the confidentiality of the info. Say even after Alice encrypts the info, it would not inherently assure that the info hasn’t been tampered with throughout transit.
Consider this situation: although Eve could also be unable to decrypt the encrypted message, they could try to switch the ciphertext in transit. This might contain altering bits, rearranging packets, or injecting malicious code, probably resulting in unintended penalties upon decryption.
This is the place a Message Detection Code (MDC) or a hash is available in image. A modification detection code (MDC) is a message digest or a checksum that may show the integrity of a message: that the message has not been modified [1].
The determine under explains how MDC is used to confirm the integrity of a message:

A Hash Function is used to generate the digest for any given message. This hash perform processes your entire content material of the message, producing a fixed-size string of characters that uniquely represents the message’s contents. This known as the message digest or MDC.
Note that any hash perform, akin to SHA-256, SHA-3, or MD5, can be utilized relying in your particular safety necessities and preferences.
Once the digest is generated, it serves as a singular fingerprint for the unique message. When Alice sends each the message and its corresponding digest to Bob, they will independently apply the identical hash perform to the obtained message. If the calculated digest matches the one obtained from Alice, it serves as irrefutable proof that the message has not undergone any modifications throughout transmission.
Message Authentication Code (MAC)
While MDC or the checksum is usually transferred over a secure channel, it could so occur that the security of the channel or the trusted social gathering itself is compromised. In such a case Eve can simply modify each the message and the digest and Bob won’t ever know if the message really got here from Alice as supposed.
What MDC lacks is a definitive assure of the message origin, leaving a possible vulnerability in confirming the true sender.
This is the place Message Authentication Code (MAC) is available in. MACs not solely make sure the integrity of the message, detecting any unauthorized alterations, however additionally they present a mechanism for authenticating the origin of the info. In different phrases, MACs supply assurance that the message is certainly originating from Alice and never by another person.
The determine under explains how MAC may also help authenticate the origin of a message apart from offering integrity examine:
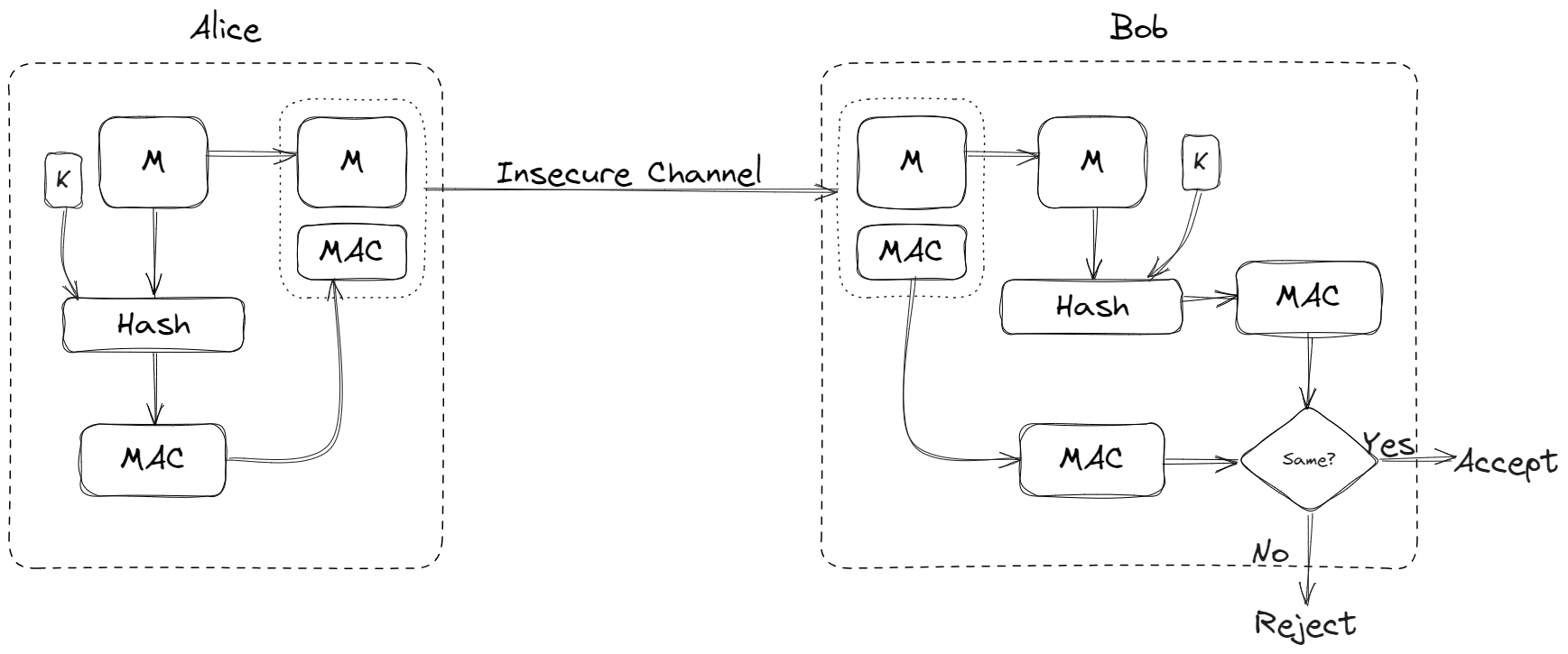
Notice that the distinction between a MDC and a MAC is that MAC additionally features a secret key (Okay) between Alice and Bob. The hash perform additionally takes in a key (Okay) together with the message (M) to generate a MAC.
[h (K | M) = MAC ]
Now each the message and MAC could be despatched over the identical insecure channel. When Bob receives this ( M + MAC ), he can separate out the message M and compute the MAC for it utilizing the identical hash perform and the key key (Okay).
Bob will then evaluate the newly computed MAC with the one he obtained. If the 2 MACs match, the message is genuine and has not been modified by an adversary.
[ Alice: S(K,M) = MAC Bob: V(M, K, MAC) = Accept/ Reject ]
Since Eve doesn’t have this secret key (Okay), they can’t modify the message and generate a legitimate MAC. Consequently, the ensuing MAC turns into a singular fingerprint, signifying not solely the integrity of the message but additionally authenticating its origin.
Hash-based MACs (HMAC)
While MAC do present a assure of authentication of the origin of a message, it’s nonetheless falls quick in guaranteeing unforgeability. It is simple for Eve to carry out a Man in The Middle (MiTM) assault, intercept the MAC + Message pair after which carry out the Length Extension Attack.
Given ( S = h( Okay || M) ) and the message (M), Eve can prolong (M) to (M’ = M || Pad || w) and create (MAC(M’)); the place (MAC(M’)) is evaluated as
( S = h( Okay || M || Pad || w) ).
Eve doesn’t require data of the key key (Okay) to increase the message (M) to (M’). When Alice receives this modified (M’) and (MAC(M’)), they’re unable to find out the modification.
HMAC or a Hash-based MAC is a particular methodology for establishing a MAC algorithm out of a collision resistant hash perform. HMAC makes use of two passes of hash computation and gives a greater immunity in opposition to size extension assaults. The determine under explains the development of HMACs.
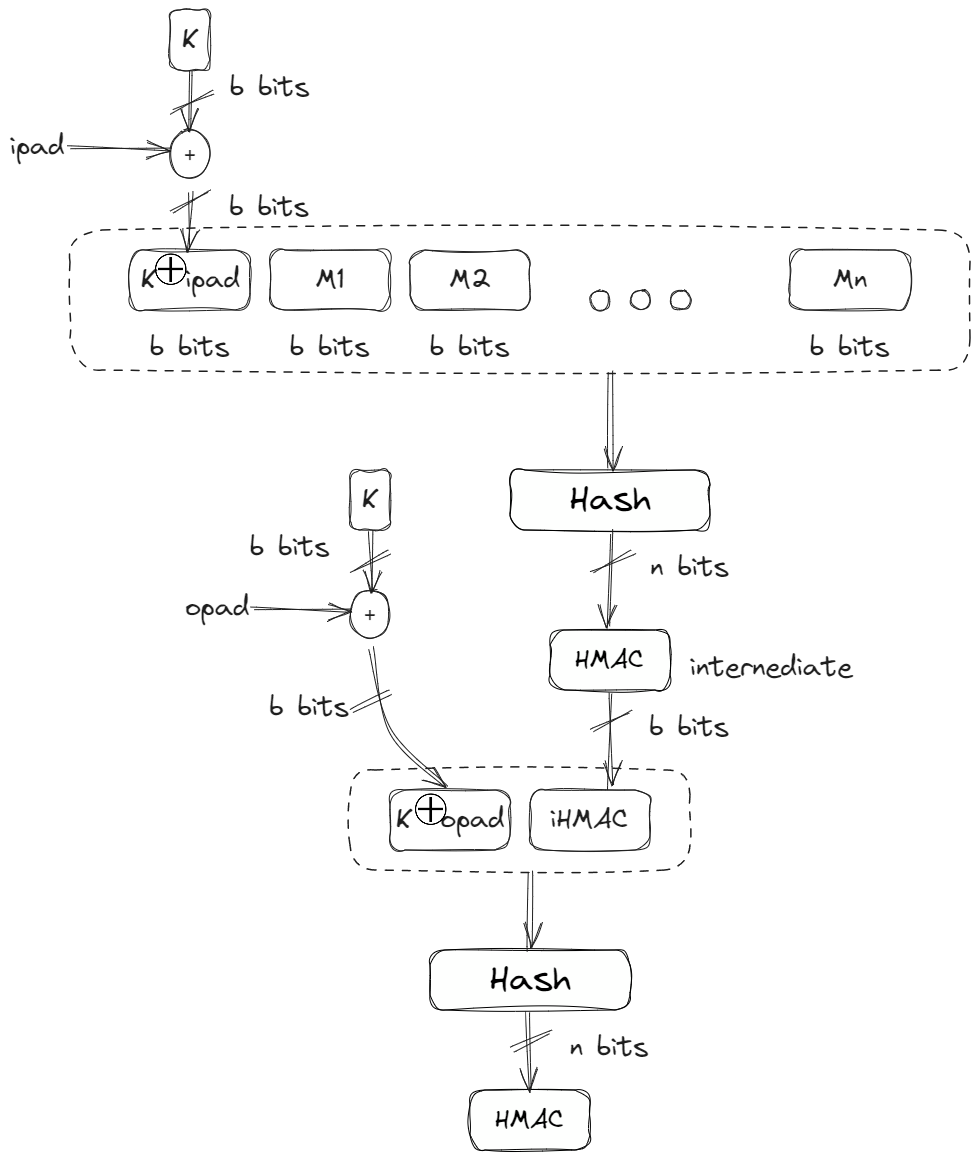
There are a number of steps concerned within the implementation of HMACs [1]:
- Divide the message into N blocks, every of b bits
- Select a secret key and left-padded with 0’s to create a b-bit key and exclusive-ored with a relentless referred to as (ipad) (enter pad).
- Use the identical secret key and XOR it with an one other fixed referred to as (opad).
The worth of (ipad) and (opad) are fastened constants as outlined within the HMAC Standards [3]. The worth of (ipad) is taken as b/8 repetition of the sequence
00110110 (hex: 36) and the worth of (opad) is taken as b/8 repetition of the sequence 01011100 (hex: 5C).
These values are outlined in such a method to have essentially the most “non-regular” Hamming distance from one another.
The Hamming distance between (ipad) and (opad) 4, which means precisely half of the bits are flipped. - Prepend the outcome produced in Step 2 to the message block. Use the hash perform on this (N+1) block to create a n bit message digest referred to as the intermediate HMAC.
- The intermediate HMAC is prepended with (0)s to make a b bit block after which the results of Step 3 is prepended to this block.
- Use the hash perform once more on the results of step 5 to get a closing n bit HMAC.
Mathematically, this may be represented as:
[ S(k, m) = H(k oplus text{opad} || H(k oplus text{ipad} || m)) ]
Now if Eve tries to increase (M) to (M’ = M || Pad || w), the ensuing HMAC development this is able to be: [ HMAC(K, M’)=H(K||opad, H(K||ipad, M || Pad || w)) ]
Due to the distinctive utility of (opad) within the outer hash, the attacker can not assemble (H(Okay||opad, <…> )) with out realizing the important thing (Okay). The outer padding disrupts the inner state for any extra enter, thwarting the attacker’s try.
The Diffie-Hellman-Merkle Protocol
One of the principle challenges in Symmetric-key Ciphers is the distribution of keys. A basic query naturally arises: How will Bob know what keys Alice has used?
A really intuitive reply to this drawback may very well be to make use of a Key Exchange or a Key Distribution Center (KDC). However, the utilization of a KDC or a Key Exchange introduces a notable caveat: the requirement of a safe channel for transmitting keys.
The safety of a system using a Key Distribution Center (KDC), akin to within the case of the Kerberos authentication protocol, is closely depending on the safety of the KDC itself. If the KDC is compromised, the cryptographic keys it manages and distributes could be uncovered, resulting in potential safety vulnerabilities all through the system as seen in a Golden Ticket Attack.
In the 12 months 1979, Ralph Merkle, Whitfield Diffie and Martin Hellman got here up with a method to Securely change Cryptographic Keys over Public Insecure Channels.
The Diffie-Hellman-Merkle Protocol gives a means for 2 events to agree upon a shared secret key over an insecure channel with out straight exchanging that key. The crypto
module in Node.js comprises the DiffieHellman
class, which is a utility for creating Diffie-Hellman key exchanges.
Before we undergo the entire capabilities outlined on this class, you will need to perceive the arithmetic that goes round in The Diffie-Hellman-Merkle Protocol. The UML Sequence Diagram under explains the steps concerned in The Diffie-Hellman-Merkle Protocol:
The course of begins with both of the social gathering who needs to ascertain a safe communication with the opposite. In this case, Alice needs to start out the communication.
Alice will first choose a randomly chosen Generator g
and a big prime quantity p
. Increasing the size of the prime quantity leads to heightened safety, because it amplifies the problem for adversaries to execute sure cryptographic assaults.
However, enlarging the prime quantity additionally comes with computational prices. Longer prime numbers require extra computational assets to carry out the important thing technology.
Now, Alice wants to pick out a Private a
and compute a modular exponentiation:
[A = g^a (text{mod} , p)]
Alice will ship over the Generator g
, the big prime p
and Alice’s Public Key A
to Bob. At this level, Bob has all of the values he wants to guage his personal modular exponentiation of:
[A = g^b (text{mod} , p)]
He will ship again this Public Key B
to Alice.
Note that up till this level, all communication are occurring over insecure channel. The values g
, p
, A
and B
“would possibly” as properly be despatched as plaintext. The Actual Secret Key is evaluated when Alice and Bob use these information to compute what is named a “Shared Secret”.
Shared Secret computed by A:
[ S = A^b (text{mod} , p) S = g^{left(abright)} (text{mod} , p) ]
Shared Secret computed by B:
[ S = B^a (text{mod} , p) S = g^{left(abright)} (text{mod} , p) ]
Notice how the Shared Secret computed by each events at their finish are the identical.
This symmetrical consequence is the essence of the Diffie-Hellman key change, the place every social gathering independently computes the shared secret utilizing their personal key and the general public key obtained from the opposite social gathering. This ensures that each Alice and Bob arrive at an equivalent Shared Secret, establishing a safe basis for additional encrypted communication.
Why is the Shared Secret Secure?
Diffie-Hellman key change depends on the mathematical rules of discrete logarithm, primitive roots and Modular exponentiation.
Modular exponentiation is the issue of computing (a^b mod n), the place (a), (b), and (n) are identified integers. Discrete logarithm is the issue of discovering (x) such that (a^x mod n = b), the place (a), (b), and (n) are identified integers and (a) is a primitive root modulo (n).
The safety of Diffie-Hellman is rooted within the computational complexity of calculating discrete logarithms.
For instance, given g
, p
and a
, it is simple to compute A
as Modular exponentiation is in P, which means that there’s a polynomial-time algorithm to unravel it.
But, the opposite means cannot be stated true. Given g
, p
, and A
, computing a
requires fixing the discrete logarithm drawback, which is broadly believed to be a computationally infeasible job [2].
Remember that each events will compute the Shared Secret at their finish and there’s no must ship over this secret to the opposite social gathering. This eliminates the chance of the Shared Secret getting intercepted by Eve and the one choice they’re left with is to unravel the discrete logarithm drawback.
Connecting the Dots
The key (Okay) that we offer in an HMAC needs to be the identical for each Alice and Bob. Now that we all know how a Diffie-Hellman-Merkle key change works, it turns into intuitive that we will plug within the shared secret as the important thing for an HMAC.
Alice can use the shared key (S) within the HMAC perform as a parameter and Bob can use the identical shared secret (S), computed at their finish, within the verification algorithm.
The crypto
module in Node.js gives numerous built-in capabilities to implement cryptographic constructs akin to HMACs and Diffie-Hellman Key Exchange. It is all the time really useful to make use of vetted cryptographic libraries and keep away from implementing cryptographic algorithms yourselves over the issues of Side Channel Attacks or a Heartbleed.
Let’s create a TypeScript/ Node.js utility to grasp the implementation and prototypes of those capabilities. The two entities concerned in communication on this utility can be Boost Inc. and Account Aggregator. Boost must ship a essential information over to the Account Aggregator.
We will first make the most of the DiffieHellman
class to create Secret Keys for each entities. Boost will then use the Secret Key to create a HMAC utilizing the Hmac
Class in Node. Account Aggregator will recieve this HMAC together with the message. They will confirm this HMAC in opposition to the newly generated HMAC from the message they obtained.
Note that the code at Account Aggregator’s finish will probably be simulated and we’ll create API endpoints for every operation to point out separation of issues on this pattern utility.
The following sequence diagram explains what the applying does:
Project Setup
In the foundation of your workspace, set up Express, Axios, sort definitions of Node, and sort definitions of Express utilizing the next command:
npm init -y | npm set up axios categorical
npm set up -D nodemon ts-node @varieties/categorical @varieties/node typescript
Configure tsconfig
as per your liking and create a file referred to as cryto.utils.ts
underneath src/utils
. Let’s create an interface and import all obligatory modules from the crypto
library:
import { createHmac, createDiffieHellman, DiffieHellman, KeyObject, BinaryLike } from 'crypto';
export interface KeyPair {
publicKey: Buffer;
personalKey: Buffer;
generator: Buffer;
prime: Buffer;
diffieHellman: DiffieHellman;
}
This interface will perform as a blueprint for managing cryptographic key pairs all through this utility. It encapsulates the private and non-private keys, generator, prime, and a Diffie-Hellman object.
By utilizing this interface we’ll guarantee a structured and standardized method to deal with cryptographic key pair data, thus selling readability and consistency in cryptographic operations inside a Node.js surroundings.
The createDiffieHellman Function
Next, we’ll outline the perform generateKeyPair
which can permit us to generate the personal and public keys, (A) and (B) together with the big prime (p) and the generator (g) utilizing the createDiffieHellman
and generateKeys
capabilities.
export perform generateKeyPair(prime?: any, generator?: any): KeyPair {
const diffieHellman = prime && generator ? createDiffieHellman(prime, 'hex', generator, 'hex') : createDiffieHellman(2048);
diffieHellman.generateKeys();
return {
publicKey: diffieHellman.getPublicKey(),
personalKey: diffieHellman.getPrivateKey(),
generator: diffieHellman.getGenerator(),
prime: diffieHellman.getPrime(),
diffieHellman,
};
}
Notice that the parameters to this perform – prime
and generator
– are elective. This is as a result of the underlying createDiffieHellman
has 5 outlined overloads:
perform createDiffieHellman(primeLength: quantity, generator?: quantity): DiffieHellman;
perform createDiffieHellman(
prime: ArrayBuffer | NodeJS.ArrayBufferView,
generator?: quantity | ArrayBuffer | NodeJS.ArrayBufferView,
): DiffieHellman;
perform createDiffieHellman(
prime: ArrayBuffer | NodeJS.ArrayBufferView,
generator: string,
generatorEncoding: BinaryToTextEncoding,
): DiffieHellman;
perform createDiffieHellman(
prime: string,
primeEncoding: BinaryToTextEncoding,
generator?: quantity | ArrayBuffer | NodeJS.ArrayBufferView,
): DiffieHellman;
perform createDiffieHellman(
prime: string,
primeEncoding: BinaryToTextEncoding,
generator: string,
generatorEncoding: BinaryToTextEncoding,
): DiffieHellman;
The first perform creates a Diffie-Hellman object with a randomly generated prime of the desired size. The createDiffieHellman(2048);
creates a Diffie-Hellman object the place the size of the randomly generated prime is 2048 bits.
When no generator worth is supplied to this perform, it takes the default worth of two. The size of the prime essentially needs to be giant and if you choose a small worth Node will throw an error signifying that this size won’t make a safe key.
Instead of passing within the size of the prime, we will cross the prime as a buffer. This is what Account Aggregator will to at their finish when Boost sends over the mandatory particulars.
Similarly you should use the opposite perform declarations as per your use case to cross the prime and generator as ArrayBuffer
or ArrayBufferView
varieties or as string
with a specified encoding.
The computeSecret Function
Now, let’s outline a technique generateSharedSecret
that takes in a Key pair and a public key as parameter and computes the shared secret (S):
export perform generateSharedSecret(keyPair: KeyPair, publicKey: Buffer): Buffer {
return keyPair.diffieHellman.computeSecret(publicKey);
}
The computeSecret
perform additionally has 4 overrides, which lets you both present the Public key parameter as string
or ArrayBufferView
in addition to choices to specify the inputEncoding
and outputEncoding
.
The createHmac Function
Now that we have computed our shared secret, let’s create a perform generateHMAC
that consumes this shared secret and generates a digest in opposition to it.
export perform generateHMAC(information: any, secretKey: KeyObject | BinaryLike): any {
information = JSON.stringify(information);
const hmac = createHmac('sha256', secretKey);
hmac.replace(information);
return hmac.digest('hex');
}
The first parameter of the createHmac
perform takes an algorithm. This is the place you must specify what underlying hash perform do you wish to use.
Remember that the safety of HMAC depends on numerous elements, together with the cryptographic energy of the underlying hash perform, the scale of its hash output, and the standard and dimension of the important thing.
The choices given to you underneath this algorithms parameter is determined by the out there algorithms supported by the OpenSSL model on the platform. To examine what algorithms can be found to you, execute the next command within the terminal:
openssl checklist -digest-algorithms
This gives you an inventory from which you’ll be able to choose your required algorithm for the underlying hash perform:
RSA-MD4 => MD4
RSA-MD5 => MD5
RSA-MDC2 => MDC2
RSA-RIPEMD160 => RIPEMD160
RSA-SHA1 => SHA1
RSA-SHA1-2 => RSA-SHA1
RSA-SHA224 => SHA224
RSA-SHA256 => SHA256
...
The secret key that the createHmac
perform takes might both be of sort KeyObject
or of sort BinaryLike
. Note that the kind BinaryLike
is a union sort in TypeScript. It is a kind that may be both a string
or a NodeJS.ArrayBufferView
.
The createHmac
perform’s information
parameter is designed to accepts strings
, Buffer
, TypedArray
and DataView
. To simplify the developer expertise and reduce complexity, we deliberately set the information
parameter sort within the generateHMAC perform as any
. Internally, we deal with the conversion to a string utilizing JSON.stringify
.
Initializing communication
Now on Boost’s finish create a file verification.controller.ts
underneath src/controllers
:
import { generateKeyPair, generateSharedSecret, generateHMAC, KeyPair } from '@enhance/v1/utils/crypto.utils';
import { KeyObject, BinaryLike } from 'crypto';
const enhanceKeyPair: KeyPair = generateKeyPair();
export perform shareKeys() {
const enhancePublicKey: Buffer = enhanceKeyPair.publicKey;
const enhancePrivateKey: Buffer = enhanceKeyPair.personalKey;
const enhanceGenerator: Buffer = enhanceKeyPair.generator;
const boostPrime: Buffer = enhanceKeyPair.prime;
const enhanceDiffieHellman = enhanceKeyPair.diffieHellman;
return {
enhancePublicKey,
enhancePrivateKey,
enhanceGenerator,
boostPrime,
enhanceDiffieHellman,
};
}
export perform hmacDigest(information: any, secretKey: KeyObject | BinaryLike): any {
return generateHMAC(JSON.stringify(information), secretKey);
}
This file imports the interface and all obligatory modules from cryto.utils.ts
and defines two wrapper capabilities – shareKeys
and hmacDigest
. shareKeys
will solely function a wrapper round generateKeyPair
which can permit builders at Boost to ship solely the required keys over to the Account Aggregator.
Setting up the Account Aggregator
At the Account Aggregator’s finish, we have to arrange a perform that computes AA’s public key and sends it over to Boost Inc. We can even want a perform to confirm the obtained HMAC of a knowledge by evaluating it in opposition to one which AA generates:
import { generateKeyPair, generateSharedSecret, generateHMAC, KeyPair } from '../utils/crypto.utils';
import axios from 'axios';
let sharedSecret: Buffer;
export async perform sendAAPublicKey(): Promise<Buffer> {
attempt {
const response = await axios.get('http://localhost:3000/init');
const enhancePublicKey: Buffer = Buffer.from(response.information.enhancePublicKey, 'hex');
const enhanceGenerator: Buffer = Buffer.from(response.information.enhanceGenerator, 'hex');
const boostPrime: Buffer = Buffer.from(response.information.boostPrime, 'hex');
const AA: KeyPair = generateKeyPair(boostPrime, enhanceGenerator);
sharedSecret = generateSharedSecret(AA, enhancePublicKey);
return AA.publicKey;
} catch (error) {
console.error('Error sending AA public key:', (error as Error).message);
throw error;
}
}
export async perform confirmData(information: any, hmac: string): Promise<string> {
attempt {
const calculatedHMAC = generateHMAC(JSON.stringify(information), sharedSecret);
return calculatedHMAC === hmac ? "Integrity and authenticity verified" : "Integrity or authenticity compromised";
} catch (error) {
console.error('Error verifying information:', (error as Error).message);
throw error;
}
}
We make an Axios request to the /init
endpoint outlined at Boost and fetch (p), (g) and (A). Once we have computed the general public key, we’ll ship that again to Boost. We can even compute our shared secret right here which we’ll use whereas verifying the HMAC within the confirmData
methodology.
Setting up the Express APIs
Now that every one the controllers and utility capabilities are in place, we’ll create a number of endpoints to facilitate communication between Boost Inc. and the Account Aggregator.
Boost:
import categorical, { Request, Response } from 'categorical';
import { hmacDigest, shareKeys } from '@enhance/v1/controllers/verification.controller';
import { KeyPair, generateSharedSecret } from '@enhance/v1/utils/crypto.utils';
import { DiffieHellman } from 'crypto';
import axios from 'axios';
const appBoost = categorical();
const PORT_BOOST = 3000;
let enhancePublicKey: Buffer, enhancePrivateKey: Buffer;
let enhanceGenerator: Buffer, boostPrime: Buffer;
let sharedSecret: Buffer;
let enhanceKeyPair: KeyPair, enhanceDiffieHellman: DiffieHellman;
appBoost.get('/init', async (req: Request, res: Response) => {
({ enhancePublicKey, enhancePrivateKey, enhanceGenerator, boostPrime, enhanceDiffieHellman } = shareKeys());
res.ship({ enhancePublicKey, enhanceGenerator, boostPrime });
});
// Simulated Data
const information = {
title: 'Boost User 1',
cellphone: '1234567890',
};
appBoost.get('/fetchData', async (req: Request, res: Response) => {
const hmac = hmacDigest(information, sharedSecret);
res.ship({ information, hmac });
});
appBoost.hear(PORT_BOOST, () => {
console.log(`Boost server is working on http://localhost:${PORT_BOOST}`);
});
The /init
endpoint, hosted by Boost, is invoked by AA inside its sendAAPublicKey
perform. When the shared secret is calculated, AA will invoke the endpoint /fetchData
to retrieve the essential data.
Account Aggregator (AA):
import categorical, { Request, Response } from 'categorical';
import { sendAAPublicKey, confirmData } from '@AA/v1/controllers/aa.controller';
import { KeyPair, generateSharedSecret } from '@enhance/v1/utils/crypto.utils';
import { DiffieHellman } from 'crypto';
import axios from 'axios';
const appAA = categorical();
const PORT_AA = 3001;
let enhancePublicKey: Buffer, enhancePrivateKey: Buffer;
let enhanceGenerator: Buffer, boostPrime: Buffer;
let AAPublicKey: Buffer;
let sharedSecret: Buffer;
let enhanceKeyPair: KeyPair, enhanceDiffieHellman: DiffieHellman;
appAA.get('/fetchAAPublicKey', async (req: Request, res: Response) => {
AAPublicKey = await sendAAPublicKey();
res.ship({ AAPublicKey: AAPublicKey.toString('hex') });
enhanceKeyPair = {
publicKey: enhancePublicKey,
personalKey: enhancePrivateKey,
generator: enhanceGenerator,
prime: boostPrime,
diffieHellman: enhanceDiffieHellman,
}
sharedSecret = generateSharedSecret(enhanceKeyPair, AAPublicKey);
});
appAA.get('/confirmData', async (req: Request, res: Response) => {
const response = await axios.get('http://localhost:3000/fetchData');
const { information, hmac } = response.information;
const verified = await confirmData(information, hmac);
res.ship({ verified });
});
appAA.hear(PORT_AA, () => {
console.log(`AA server is working on http://localhost:${PORT_AA}`);
});
The fetchAAPublicKey
endpoint, hosted as AA’s finish, will probably be invoked by Boost when it needs to guage the Shared Secret. The confirmData
methodology is encapsulated inside a GET
request, enabling both social gathering to verify the integrity of the transmitted information.
Invoking the APIs
Head over to your Postman Client to check out these APIs. Since the sendAAPublicKey
methodology takes care of the initiation, we have to begin our communication utilizing the /fetchAAPublicKey
endpoint:

You will observe the AA’s public key as a response. Now, Boost Inc. will use this Public Key and consider the Shared Secret.
Once that’s completed, it’ll use the Shared Secret to compute the message digest within the /fetchData
endpoint. Since /confirmData
invokes the previous endpoint, we’ll examine this in motion on our Postman Client:

You will discover that the /confirmData
response declares the profitable verification of each integrity and authenticity. This acknowledgment ensures that the transmitted information stays untampered and originates from the authenticated supply, offering a layer of safety for communication between the 2 entities.
Wrapping Up
And there you have got it: by using HMACs and the Diffie-Hellman-Merkle Key Exchange, you may confirm the integrity and authenticity of your transmitted information, enhancing the safety of your functions and guaranteeing a dependable API communication framework for builders.
By understanding the intricacies and mathematical underpinnings of those practices, now you can make knowledgeable selections, fortifying your system in opposition to tampering threats.
Find the whole code snippets right here — GitHub Gist | HamdaanAliQuatil.
You could discover me on X (previously Twitter) – Hamdaan Ali Quatil.
References
[1] Behrouz A. Forouzan – Introduction to Cryptography and Network Security
[2] New Directions in Cryptography, Whitfield Diffie and Martin E. Hellman diffie.hellman.pdf (jhu.edu)
[3] Keying Hash Functions for Message Authentication, Mihir Bellare, Ran Canetti, Hugo Krawczyk https://cseweb.ucsd.edu/~mihir/papers/kmd5.pdf