Things on the web are all the time altering. Sometimes web sites themselves change. Sometimes issues on an internet site dynamically change values in JavaScript when you’re interacting with it. This is the place my curiosity peaks.
I’ve all the time seen little issues like this in life and puzzled the way it’s occurring behind the scenes. I can stroll as much as a merchandising machine, put in no matter loopy amount of cash they’re charging today, select, and the machine delivers my desired snack… if their {hardware} is working after all.
Become a Full Stack JavaScript Developer Job in 2024!
Learn to code with Treehouse Techdegree’s curated curriculum stuffed with real-world tasks and alongside unimaginable pupil assist. Build your portfolio. Get licensed. Land your dream job in tech. Sign up for a free, 7-day trial right this moment!
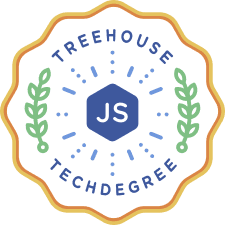
Luckily for us, in JavaScript, that is very simple to do. We can change the worth of 1 factor primarily based on one other and we are able to achieve this in real-time. This turns into particularly useful when working with types.
Now that I’ve doughnuts on my thoughts, let’s persist with this merchandising machine concept and simplify it to an HTML kind for this instance. Let’s think about there are two machines facet by facet: one containing drinks and one containing snacks.
Setting the Stage with HTML
Here’s my fundamental HTML. I’ve a kind factor that accommodates a choose factor on the high. This is the place we determine which machine to strategy, drinks or snacks. Below that’s one other choose factor with all our drinks and snack choices. I’ve utilized an information attribute to those to distinguish them inside our JavaScript later. Finally, under that, we’ve a submit button.
<kind>
<choose identify="machineSelect" id="machineSelect">
<possibility worth="default" chosen hidden disabled>
Select a Machine
</possibility>
<possibility worth="drinks">Drinks</possibility>
<possibility worth="snacks">Snacks</possibility>
</choose>
<choose identify="merchandiseSelect" id="merchandiseSelect">
<possibility worth="default" chosen hidden disabled>Select an Item</possibility>
<possibility data-category="drinks" worth="water">Water</possibility>
<possibility data-category="drinks" worth="soda">Soda</possibility>
<possibility data-category="snacks" worth="chips">Chips</possibility>
<possibility data-category="snacks" worth="donuts">Donuts</possibility>
</choose>
<button sort="submit">Purchase</button>
</kind>
Bringing the Form to Life with JavaScript
So, what’s our purpose right here? We need to, in real-time, solely present related gadgets in our second choose menu primarily based on what the client has chosen within the first. It wouldn’t make sense in our case to even have two completely different machines in the event that they each bought drinks and snacks, irrespective of how a lot gremlin labor that will save.
First issues first, in our JavaScript, we want retailer reference to a couple key components that we are able to entry over time: every of our choose components and every of the merchandise choices throughout the second menu. I’ll retailer the primary two in variables utilizing the questionSelector technique. Notice for the choices, I’m utilizing questionSelectorAll, grabbing the choices straight from the merchandiseSelect factor and provided that they’ve that data-category attribute. This is as a result of I’m storing multiple factor, I solely need choices nested inside that particular factor, and I need to keep away from getting reference to the primary default possibility. I’d additionally prefer to disable the merchandiseSelect menu upon loading the web page. This will probably be our little method of stopping the wandering toddler from hitting buttons and getting free snacks.
// retailer our needed components in variables
const machineSelect = doc.questionSelector("#machineSelect");
const merchandiseSelect = doc.questionSelector("#merchandiseSelect");
const itemOptions = merchandiseSelect.questionSelectorAll("possibility[data-category]");
// disable the merchandise menu upon web page load
merchandiseSelect.disabled = true;
Alright, so within the occasion of adjustments occurring to the machineSelect, we have to add a way to hear for that change. This is very simple through the use of JavaScript’s addEventListener technique and specifying the “change” occasion… see what I did there? I’ll see myself out shortly.
We can then go it an nameless operate utilizing the arrow syntax and add our code inside.
// hear for adjustments on the machine menu
machineSelect.addEventListener("change", () => {
// code we need to run on each change
});
The very first thing we must always do is allow our merchandiseSelect factor to allow them to work together with it now. The proud gremlin grants permission for the consumer to pick out an merchandise.
// allow the merchandise menu
merchandiseSelect.disabled = false;
Dynamically Change Value Based on User’s Choice
Now we’ve hit our problem. Currently, all our merchandise choices seem when opening the second menu, even when they don’t correlate to the kind of machine chosen, permitting room for consumer errors in our kind.
We must loop over our itemOptions, examine what its data-category worth is, and conceal the choices that don’t match up. We can use just a few several types of loops right here, however I desire a forEach loop since I do know I’m going to must iterate over each merchandise each time, and I desire the easy syntax.
// loop over every merchandise possibility
itemOptions.forEach((merchandise) => {
}
The very first thing I would like is the at present iterated-over merchandise’s data-category worth. We can use the getAttribute technique to perform this and retailer the returned worth right into a variable.
itemOptions.forEach((merchandise) => {
// retailer the merchandise's class worth
const class = merchandise.getAttribute("data-category");
}
If you look again at our HTML, you’ll discover I’ve purposefully given the data-category’s values the identical strings because the values on our machineSelect’s choices. This makes the subsequent step handy for me, however in the event you don’t have this type of management over what you’re engaged on, you might must take an additional step in evaluating these values.
Now looks as if a good time for a conditional assertion. With this setup, we are able to merely examine if our new variable will not be strictly equal to the machineSelect’s worth. If this situation is true, which means they don’t match up, we merely cover this present possibility. I’ve additionally added the disabled attribute as a result of some browsers like being completely different and troublesome for us devs *ahem* Safari *cough sound*. If the consumer is indecisive whether or not they’re hungry or thirsty and make a number of adjustments to the machineSelect, we have to be sure that beforehand hidden and disabled choices are seen and enabled once more within the else block.
// cover non-matching choices, show matching ones
if (class !== machineSelect.worth) {
merchandise.hidden = true;
merchandise.disabled = true;
} else {
merchandise.hidden = false;
merchandise.disabled = false;
}
Awesome! Only our related gadgets seem as choices within the second choose menu!
Become a Full Stack JavaScript Developer Job in 2024!
Learn to code with Treehouse Techdegree’s curated curriculum stuffed with real-world tasks and alongside unimaginable pupil assist. Build your portfolio. Get licensed. Land your dream job in tech. Sign up for a free, 7-day trial right this moment!
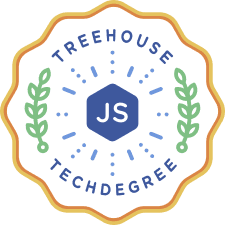
But beware! There remains to be a chance of a really sneaky concern that may trigger a consumer error right here. If, for instance, I select the “Drinks” machine after which choose “Soda” as an merchandise, then my abdomen growls and I understand I solely manage to pay for for one merchandise and I ought to most likely get some doughnuts as an alternative, and change to the “Snacks” machine, our gadgets menu nonetheless has our “Soda” choice. This clearly couldn’t occur in actual life after all, however this leaves room for me to submit this kind with a mismatched choice. This might confuse and anger our gremlin buddy.
Resetting Choices for Consistency
It could be ultimate if each time the consumer adjustments the machine, the merchandiseSelect menu both: adjustments again to the default possibility, or we assign an identical possibility as a default. I’ll go together with the primary possibility so it’s apparent they should make a brand new choice and don’t accidently submit with an possibility I supplied.
So, after our loop, we are able to merely assign our merchandiseSelect’s worth to the worth of our default possibility, which in the event you look again on the HTML, I’ve made it the string “default”.
// reset the worth of the merchandise menu
merchandiseSelect.worth = "default";
Now, every time the machine adjustments, our merchandise menu is introduced again to the default chosen possibility. Nice!
Here’s the JavaScript in entire.
// retailer our needed components in variables
const machineSelect = doc.questionSelector("#machineSelect");
const merchandiseSelect = doc.questionSelector("#merchandiseSelect");
const itemOptions = merchandiseSelect.questionSelectorAll("possibility[data-category]");
// disable the merchandise menu upon web page load
merchandiseSelect.disabled = true;
// hear for adjustments on the machine menu
machineSelect.addEventListener("change", () => {
// allow the merchandise menu
merchandiseSelect.disabled = false;
// loop over every merchandise possibility
itemOptions.forEach((merchandise) => {
// retailer the merchandise's class worth
const class = merchandise.getAttribute("data-category");
// cover non-matching choices, show matching ones
if (class !== machineSelect.worth) {
merchandise.hidden = true;
merchandise.disabled = true;
} else {
merchandise.hidden = false;
merchandise.disabled = false;
}
});
// reset the worth of the merchandise menu
merchandiseSelect.worth = "default";
});
I’ll depart it at this, however I encourage you to get some follow in and increase on this additional! You can add an eventListener on the shape itself and hear for the “submit” occasion and let the consumer know you’re sorry, however the gremlin ate your snack already, however thanks for the cash. Or you possibly can implement some validation, guaranteeing the consumer has made an merchandise choice.
You might add in one other choose menu or enter discipline to see how a lot cash the consumer is inserting, apply a value to every merchandise and calculate the change that the gremlin will most likely pocket anyway.
The machineSelect might be radio buttons as an alternative of a choose factor. The sky’s the restrict in the case of snacks… I imply JavaScript!
This additionally doesn’t must be carried out solely in types, after all. You can hear for all types of occasions on all types of components, get values, set different values, and produce your webpages to life! I hope this proves useful for any person, I’m off to seek out some doughnuts. Happy coding everybody!
Boost Your Coding Skills: Start Your Free 7-Day Trial
Ready to take your coding abilities to the subsequent degree? Sign up now for a free 7-day trial and unlock limitless entry to our intensive library of coding sources, tutorials, and tasks. Whether you’re a newbie or an skilled developer, there’s all the time one thing new to be taught.
Don’t miss out on this chance to increase your data and develop your coding experience. Join us right this moment and begin your journey in direction of mastering coding!