Ever since I used to be a child, I’ve been hooked on video video games. My first expertise with coding concerned form of a wild challenge—a Tron races simulator in Quick Basic on an historical IBM laptop (I used to be like 6 years outdated, so I did not actually understood what I used to be doing).
Fast ahead via years of coding and right here I’m, nonetheless writing software program and going into the world of recreation improvement.
With my sights set on turning into an indie recreation developer, I made a decision to discover PyGame, given my “fluency” in Python with instructing it and all.
After some brainstorming, I landed on a unusual idea: a recreation the place you play as an alien cruising round in a UFO. Your mission? Hit a particular abduction quota every week to dodge sanctions from the Intergalactic Federation.
I will not lie, I used to be closely impressed by the outdated “Luna lander” that I had in the identical outdated IBM laptop.
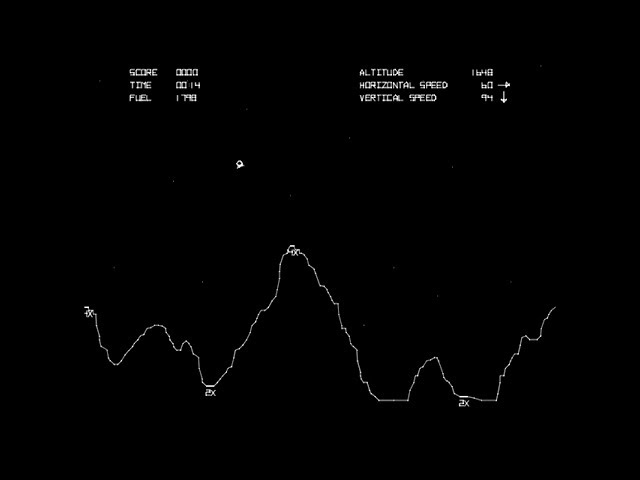
Why I believe making video games is cooler than your common coding challenge
So, think about you are coding, and abruptly it hits you – video games! Yep, we’re speaking concerning the stuff that turns a display into an interactive universe the place you are not only a coder – you are a wizard creating spells (or traces of code) that make issues transfer, shoot, and explode with out authorized repercussions.
Here’s why diving into recreation improvement is like discovering the key degree into software program improvement:
- It’s coding with a objective: Forget boring applications – you are making a universe the place aliens abduct cows as a result of…why not?
- No extra lone wolf coding: Games want greater than code. They additionally want tales, characters, and funky graphics. Suddenly, you are not only a coder – you are a one-person military conquering design, storytelling, and perhaps a little bit of sound engineering.
- It’s like LEGO for coders: You get to construct stuff, break it, after which construct it once more! Game improvement is all about trial and error, making it the LEGO of coding initiatives. Who stated debugging cannot be enjoyable?
- Creativity occasion: Ever needed to create a world the place gravity works sideways, and cows put on spacesuits? In recreation improvement, your creativity runs wild.
- Solving mysteries, coding version: Games include puzzles. Not only for gamers, however for you, the mastermind behind the scenes. Think of it as creating your personal coding thriller novel the place each bug is a plot twist ready to be solved.
- Learn by taking part in: Remember these boring textbooks? Well, neglect them. With recreation improvement, you are not simply studying – you are taking part in.
- Show off your expertise: Building video games is not nearly enjoyable. It’s about flexing your coding muscle mass. Your recreation is your trophy, your badge of honor. Imagine displaying as much as a job interview with a recreation you constructed. Who’s cool now?
So, why accept coding when you may create a universe? Game improvement is the place coding turns into an epic journey, and also you, my good friend, are the hero. Ready to degree up?
Alright, let’s craft this recreation collectively, step-by-step, and make it a blast! Wondering what it will appear like when it is all finished? Check out this snazzy preview:
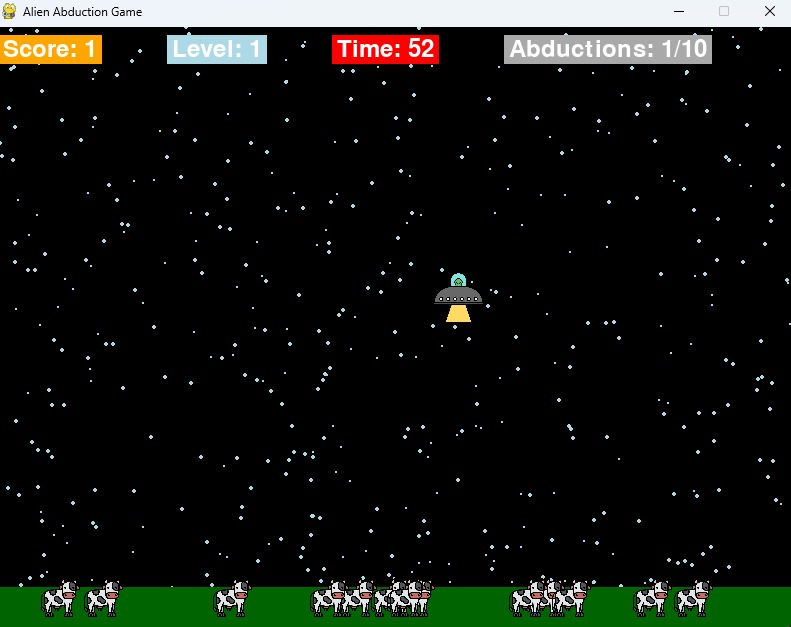
Table of Contents:
How to Set Up the Environment
Install PyGame
Let’s kick off this journey by establishing the playground. First, you will want to put in PyGame. Here’s a easy information to get you began.
1. Create a brand new listing
Open your favourite terminal and navigate to the situation the place you wish to maintain your recreation. Create a brand new listing on your challenge. Let’s name it “AlienAbductionGame.“
mkdir AlienAbductionGame
2. Navigate to the challenge listing
Move into your newly created listing.
cd AlienAbductionGame
3. Install PyGame
pip set up pygame
Great! Now you are armed with PyGame, and also you’re able to get into the coding.
What else do it is advisable to begin?
You have already got Python with PyGame put in in your laptop. So, the very last thing you want is your favourite code editor. I’ll use and suggest Visual Studio Code, however you should use some other editor that you simply like or really feel comfy with.
Once you have got every thing put in and your editor prepared, let’s begin by understanding the sport idea and its mechanics.
How to Design the Game Concept
Game thought: Alien abduction journey
Picture this: You’re an alien pilot cruising via the cosmos in your trusty UFO. Unfortunately, you are falling behind in your weekly quota of abductions, and the Intergalactic Federation is not too happy. To keep away from a cosmic penalty, you need to abduct varied targets on Earth.
Mechanics
Abduction quota and ranges:
At the beginning of every degree, you are given a particular abduction quota. This represents the variety of cows you need to abduct to progress.
The recreation consists of ten ranges, every with an growing quota. Completing one degree unlocks the subsequent and restart the quota counter.
UFO Controls:
Use the arrow keys or your most popular controls to navigate the UFO throughout the sport display.
Precise actions are essential for profitable abductions. Mastering the controls ensures environment friendly goal acquisition.
Targets – lovely cows:
Targets are charming, pixelated cows wandering on the Earth’s floor. You’ll method a cow, activate your tractor beam (area bar), and watch the cow dissappear out of your display.
Tractor beam mechanics:
You’ll press the area bar to activate the tractor beam, which extends out of your UFO to the bottom.
When the beam touches a cow, it triggers the kidnapping course of, and the cow dissappears, growing your rating and abduction quota.
Timer and urgency:
Each degree comes with a countdown timer, including a way of urgency to your abductions.
Successfully reaching the kidnapping quota earlier than the timer hits zero ensures development to the subsequent degree.
How to determine on recreation parts (spaceship, targets, and so forth)
When it involves choosing your recreation parts, simplicity is vital. The less complicated, the higher. So, let’s check out what you will have to make the sport mechanics work.
- Spaceship (UFO):
- Instead of intricate designs, concentrate on a particular UFO sprite, a cute one
- Prioritize easy actions and responsiveness to participant controls.
2. Targets (cows):
- Opt for a cute cow sprite that aligns with the sport’s humor.
- Consider altering the cow’s colour when it is kidnapped – you’ll code a purple bubble signifying abduction.
3. Background and atmosphere:
- Maintain a clear and simple background, adjusting the colour scheme to match the sport’s development.
- Gradually modify the star colour with every degree to suggest development.
4. Tractor beam:
- Ensure the tractor beam is visually distinguishable. You can experiment with a fundamental, but efficient, plain yellow colour.
5. Level indicators:
- Implement a easy degree indicator displaying the present degree. This could be a small part on the nook of the display.
6. Game Over and victory screens:
- Design easy screens indicating success or failure. A easy “Game Over” and “You Win” with accompanying textual content will suffice, like the next one
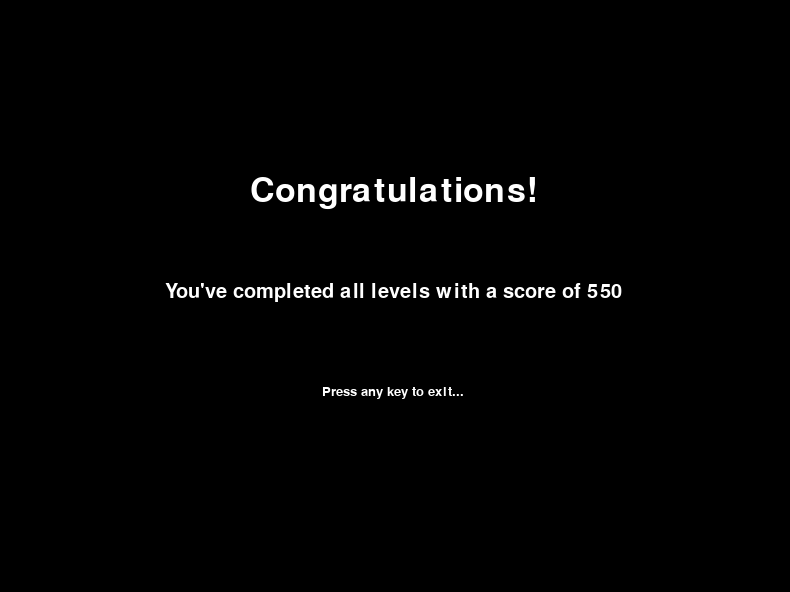
How to Code the Game
Walkthrough of key sections of the code
Now that you’ve got a transparent idea and understanding of our recreation mechanics and it is parts, it is time to get into the code. We’ll stroll via the important thing sections of the code.
First, let’s begin by importing the stuff it is advisable to code our recreation:
import pygame
import random
import sys
In this part, you might be importing three modules: pygame
, random
, and sys
.
- pygame: This is the primary library you are utilizing to create the sport. It supplies capabilities and instruments for dealing with graphics, person enter, and extra.
- random: you will use this module to generate random numbers. This turns out to be useful while you need issues to occur unpredictably, just like the preliminary place of targets.
- sys: This module supplies entry to some variables used or maintained by the Python interpreter and to capabilities that work together strongly with the interpreter. You’ll use it for dealing with system occasions, akin to quitting the sport when the participant closes the window.
So, you are mainly gearing up with the instruments it is advisable to make the sport visually interesting, dynamic, and conscious of participant enter.
How to code the beginning display
def start_screen(display):
# Constants
WIDTH, HEIGHT = 800, 600
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
FONT_SIZE = 30
# Font for displaying the textual content
font = pygame.font.Font(None, FONT_SIZE)
# Fill the display with the background colour
display.fill(BLACK)
# Introduction textual content
intro_text = [
"Welcome, Alien Abductor!",
"You're behind on your weekly quota of abductions.",
"Help the alien catch up by abducting targets on Earth!",
"",
"----------------------------------------------------------------------------------------------",
"Move the UFO with ARROWS and",
"press SPACE to abduct cows with the track bean.",
"----------------------------------------------------------------------------------------------",
"",
"Press any key to start the game...",
"",
]
# Render and show the introduction textual content
y_position = HEIGHT // 4
for line in intro_text:
textual content = font.render(line, True, WHITE)
text_rect = textual content.get_rect(middle=(WIDTH // 2, y_position))
display.blit(textual content, text_rect)
y_position += FONT_SIZE
pygame.show.flip()
# Wait for a key press to start out the sport
wait_for_key()
def wait_for_key():
ready = True
whereas ready:
for occasion in pygame.occasion.get():
if occasion.kind == pygame.QUIT:
pygame.stop()
sys.exit()
elif occasion.kind == pygame.KEYDOWN:
ready = False
Code rationalization:
- The
start_screen
perform takes adisplay
parameter, which is the Pygame window the place you will show our introduction. It units up some constants for display dimensions, colours, and font measurement. - The display is full of a black background.
- An introduction textual content is outlined in
intro_text
, offering a welcome message, directions, and a immediate to start out the sport. - Using Pygame’s font performance, the textual content is rendered and displayed on the display.
- The perform then waits for a key press to start out the sport by calling the
wait_for_key
perform. - The
wait_for_key
perform loops till a secret is pressed or the person closes the window. If a secret is pressed, the loop exits, and the introduction display disappears.
So, by now, it’s best to have one thing like this:
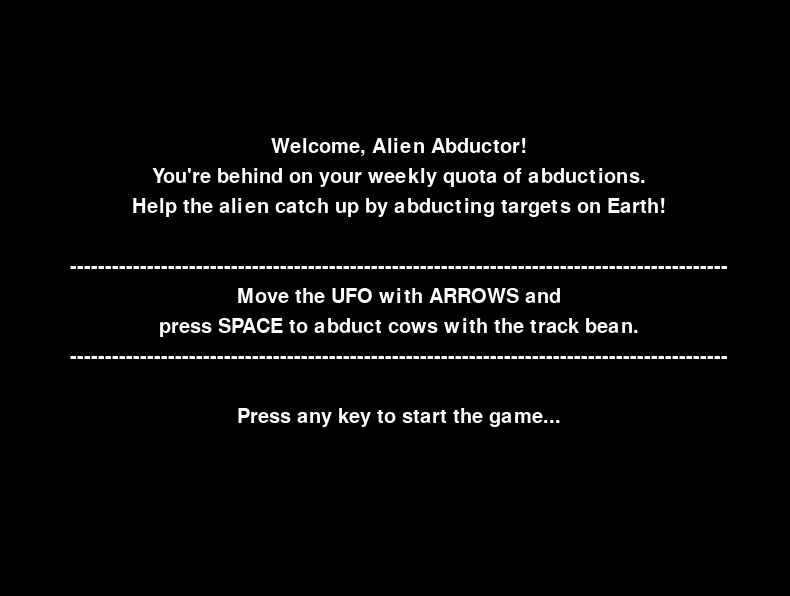
How to construct textual content on the display perform
Now, let’s take a look at the next block of code:
def show_text_on_screen(display, textual content, font_size, y_position):
font = pygame.font.Font(None, font_size)
text_render = font.render(textual content, True, (255, 255, 255))
text_rect = text_render.get_rect(middle=(WIDTH // 2, y_position))
display.blit(text_render, text_rect)
This code defines a perform show_text_on_screen
that shows a textual content message on the Pygame window. Let’s break it down:
- The perform takes 4 parameters:
display
: The Pygame window the place the textual content can be displayedtextual content
: The message or textual content string to be displayed.font_size
: The measurement of the font for the textual content.y_position
: The vertical place on the display the place the textual content can be centered.
- It creates a Pygame font object with the required measurement.
- Using the font object, it renders the textual content with white colour (
(255, 255, 255)
represents RGB values for white). - The
get_rect
technique is used to get the oblong space that encloses the rendered textual content. The middle of this rectangle is ready to be on the horizontal middle and the required vertical place. - Finally, the rendered textual content is blitted (drawn) onto the display on the specified place.
- This perform supplies a handy strategy to show textual content messages on the display with a specified font measurement and vertical place.
How to code the “Game Over” display
Now, you’ll code a recreation over display, so maintain going and examine the subsequent block of code:
def game_over_screen(display):
display.fill((0, 0, 0)) # Fill the display with black
show_text_on_screen(display, "Game Over", 50, HEIGHT // 3)
show_text_on_screen(display, f"Your ultimate rating: {rating}", 30, HEIGHT // 2)
show_text_on_screen(display, "Press any key to exit...", 20, HEIGHT * 2 // 3)
pygame.show.flip()
wait_for_key()
Picking aside the sport over display:
display.fill((0, 0, 0))
: Fills the complete Pygame window with a black background.show_text_on_screen(display, "Game Over", 50, HEIGHT // 3)
: Displays the textual content “Game Over” with a font measurement of fifty, centered vertically at one-third of the display peak.show_text_on_screen(display, f"Your ultimate rating: {rating}", 30, HEIGHT // 2)
: Displays the participant’s ultimate rating with a font measurement of 30, centered vertically at half of the display peak.show_text_on_screen(display, "Press any key to exit...", 20, HEIGHT * 2 // 3)
: Displays the instruction to press any key to exit with a font measurement of 20, centered vertically at two-thirds of the display peak.pygame.show.flip()
: Updates the show to point out the adjustments.wait_for_key()
: Waits for a key press earlier than continuing, successfully making the display keep till the participant interacts.
This perform is used to point out a recreation over display with related info akin to the ultimate rating and an exit instruction, one thing that by now ought to appear like this:

You win! How to code the victory display 🙂
And final however not least, you have got the victory display, so let’s check out the display code:
def victory_screen(display):
display.fill((0, 0, 0)) # Fill the display with black
show_text_on_screen(display, "Congratulations!", 50, HEIGHT // 3)
show_text_on_screen(display, f"You've accomplished all ranges with a rating of {rating}", 30, HEIGHT // 2)
show_text_on_screen(display, "Press any key to exit...", 20, HEIGHT * 2 // 3)
pygame.show.flip()
wait_for_key()
Code rationalization:
display.fill((0, 0, 0))
: Fills the complete Pygame window with a black background.show_text_on_screen(display, "Congratulations!", 50, HEIGHT // 3)
: Displays the textual content “Congratulations!” with a font measurement of fifty, centered vertically at one-third of the display peak.show_text_on_screen(display, f"You've accomplished all ranges with a rating of {rating}", 30, HEIGHT // 2)
: Displays a congratulatory message with the participant’s ultimate rating and a font measurement of 30, centered vertically at half of the display peak.show_text_on_screen(display, "Press any key to exit...", 20, HEIGHT * 2 // 3)
: Displays the instruction to press any key to exit with a font measurement of 20, centered vertically at two-thirds of the display peak.pygame.show.flip()
: Updates the show to point out the adjustments.wait_for_key()
: Waits for a key press earlier than continuing, successfully making the display keep till the participant interacts.
This perform is used to point out a victory display with a congratulatory message and the participant’s ultimate rating.
So now you have got the beginning of the sport, the screens you’ll use to speak with the participant.
Let’s construct some sprites
Next up, you’ll code the bottom parameters such because the window measurement, sprites (the UFO and cow), colours, stars, and so forth.
Take a glance to the next block of code:
# Load spaceship and cow photographs
ovni = pygame.picture.load("ovni.png")
cow = pygame.picture.load("cow.png")
# Resize photographs if wanted
ovni = pygame.remodel.scale(ovni, (50, 50))
cow = pygame.remodel.scale(cow, (40, 40))
# Initialize Pygame
pygame.init()
# Constants
WIDTH, HEIGHT = 800, 600
FPS = 60
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
YELLOW = (255, 255, 0)
GRAY = (169, 169, 169)
ORANGE = (255, 165, 0)
LIGHT_BLUE = (173, 216, 230) # Light blue colour for the extent indicator
SHIP_GREEN = (0, 255, 0) # Green colour for the ship
GRASS_GREEN = (0, 100, 0) # Darker inexperienced colour for the grass
STAR_COUNT = int(WIDTH * HEIGHT * 0.001)
Code rationalization:
ovni = pygame.picture.load("ovni.png")
andcow = pygame.picture.load("cow.png")
: Load the spaceship (ovni) and cow photographs from recordsdata.ovni = pygame.remodel.scale(ovni, (50, 50))
andcow = pygame.remodel.scale(cow, (40, 40))
: Resize the photographs if wanted. In this case, the spaceship is resized to a width and peak of fifty pixels, and the cow is resized to a width and peak of 40 pixels.pygame.init()
: Initializes Pygame.
Constants:
WIDTH, HEIGHT = 800, 600
: Sets the width and peak of the sport window.FPS = 60
: Sets the frames per second for the sport.- Colors:
BLACK, WHITE, RED, YELLOW, GRAY, ORANGE
: Define colour constants utilizing RGB values.LIGHT_BLUE, SHIP_GREEN, GRASS_GREEN
: Additional colour constants for particular parts. STAR_COUNT
: Calculates the variety of stars based mostly on the window measurement.
This part handles the setup of photographs for the spaceship and cow, adjusting their sizes as wanted. It additionally establishes constants for varied elements of the sport.
The subsequent steps contain creating the sport window and implementing participant controls, collision detection, and different important parts of the gameplay.
How to code the sport window and arrange the FPS
# Create the sport window
display = pygame.show.set_mode((WIDTH, HEIGHT))
pygame.show.set_caption("Alien Abduction Game")
# Clock to manage the body fee
clock = pygame.time.Clock()
# Player (alien spaceship)
player_rect = pygame.Rect(WIDTH // 2 - 25, 10, 50, 50)
player_speed = 5
# List to retailer targets (animals)
targets = []
# Set preliminary rating
rating = 0
# Font for displaying the rating, degree, and timer
font = pygame.font.Font(None, 36)
# Flag to trace if spacebar is pressed
space_pressed = False
# List to retailer stars
stars = [{'x': random.randint(0, WIDTH), 'y': random.randint(0, HEIGHT), 'size': random.randint(1, 3),
'color': LIGHT_BLUE} for _ in range(STAR_COUNT)]
# Grassy space on the backside
grass_rect = pygame.Rect(0, HEIGHT - 40, WIDTH, 40)
# Level and Countdown Variables
current_level = 1
abduction_target = 10 # Initial goal
countdown_timer = 60 # Initial countdown timer in seconds
current_score = 0 # New counter to trace the rating for the present degree
# Counter to manage the tempo of goal appearances
target_spawn_counter = 0
TARGET_SPAWN_RATE = max(30, 120 - (current_level * 90)) # Adjust the speed based mostly on the present degree
# List of colours for every degree
level_colors = [
LIGHT_BLUE,
ORANGE,
RED,
YELLOW,
GRAY,
(0, 255, 0), # Green
(255, 0, 255), # Purple
(0, 255, 255), # Cyan
(255, 165, 0), # Orange
(128, 0, 128), # Indigo
]
Code rationalization:
Creating the Game Window:
display = pygame.show.set_mode((WIDTH, HEIGHT))
: This line creates the sport window with the required width and peak.pygame.show.set_caption("Alien Abduction Game")
: Sets the title or caption of the sport window.
Clock for Frame Rate:
clock = pygame.time.Clock()
: Initializes a clock object to manage the body fee of the sport.
Player (Alien Spaceship):
player_rect = pygame.Rect(WIDTH // 2 - 25, 10, 50, 50)
: Defines an oblong space for the participant (alien spaceship) on the high middle of the window.player_speed = 5
: Sets the pace at which the participant can transfer.
Targets (Animals):
targets = []
: Initializes an empty checklist to retailer targets (animals).
Score:
rating = 0
: Initializes the rating to zero.
Font for Displaying Text:
font = pygame.font.Font(None, 36)
: Creates a font object for displaying rating, degree, and timer.
Spacebar Pressed Flag:
space_pressed = False
: Initializes a flag to trace whether or not the spacebar is pressed.
Stars:
stars = [...]
: Creates an inventory of stars with random positions, sizes, and colours. This is used to create a starry background.
Grassy Area on the Bottom:
grass_rect = pygame.Rect(0, HEIGHT - 40, WIDTH, 40)
: Defines an oblong space on the backside for a grassy background.
Level and Countdown Variables:
current_level = 1
: Initializes the sport at degree 1.abduction_target = 10
: Sets the preliminary abduction goal.countdown_timer = 60
: Sets the preliminary countdown timer in seconds.current_score = 0
: Initializes a counter to trace the rating for the present degree.
Target Spawn Counter:
target_spawn_counter = 0
: Initializes a counter to manage the tempo of goal appearances.
Target Spawn Rate:
TARGET_SPAWN_RATE = max(30, 120 - (current_level * 90))
: Adjusts the speed of goal look based mostly on the present degree.
Colors for Each Level:
level_colors = [...]
: Defines an inventory of colours for every degree, which can be used within the recreation.
These variables and settings set up the preliminary state of the sport, defining the participant, targets, scoring system, and visible parts. Your recreation will evolve and reply to participant actions based mostly on these preliminary circumstances.
How to code the sport mechanisms
Take a take a look at these capabilities that you’ll use to run the sport. Try to code it your self earlier than you copy and paste the code. This will aid you perceive the pondering course of between the mechanics and the precise code.
# Function to show the beginning display
start_screen(display)
# Main recreation loop
working = True
game_started = False # Flag to trace whether or not the sport has began
whereas working:
for occasion in pygame.occasion.get():
if occasion.kind == pygame.QUIT:
working = False
elif occasion.kind == pygame.KEYDOWN:
if game_started:
game_started = True # Set the flag to True to keep away from calling start_screen repeatedly
proceed # Skip the remainder of the loop till the sport has began
elif occasion.key == pygame.K_SPACE:
space_pressed = True
elif occasion.kind == pygame.KEYUP and occasion.key == pygame.K_SPACE:
space_pressed = False
keys = pygame.key.get_pressed()
# Move the participant
player_rect.x += (keys[pygame.K_RIGHT] - keys[pygame.K_LEFT]) * player_speed
player_rect.y += (keys[pygame.K_DOWN] - keys[pygame.K_UP]) * player_speed
# Ensure the participant stays throughout the display boundaries
player_rect.x = max(0, min(player_rect.x, WIDTH - player_rect.width))
player_rect.y = max(0, min(player_rect.y, HEIGHT - player_rect.peak))
# Spawn a brand new goal based mostly on the counter
target_spawn_counter += 1
if target_spawn_counter >= TARGET_SPAWN_RATE:
target_rect = pygame.Rect(random.randint(0, WIDTH - 20), HEIGHT - 50, 50, 50)
targets.append(target_rect)
target_spawn_counter = 0
# Update star glow animation and colour for the present degree
for star in stars:
star['size'] += 0.05
if star['size'] > 3:
star['size'] = 1
star['color'] = level_colors[current_level - 1]
Code rationalization:
Display Start Screen:
start_screen(display)
: Calls thestart_screen
perform to show the preliminary begin display.
Main Game Loop:
working = True
: Initializes a flag to manage the primary recreation loop.game_started = False
: Initializes a flag to trace whether or not the sport has began.
Event Handling:
- The loop iterates via pygame occasions to examine for person enter and window occasions.
pygame.QUIT
: If the person closes the sport window, theworking
flag is ready toFalse
, exiting the sport.pygame.KEYDOWN
: If a secret is pressed:- If the sport has began (
game_started
isTrue
), the loop is skipped, stopping repeated calls to the beginning display. - If the spacebar (
pygame.K_SPACE
) is pressed and the sport hasn’t began,space_pressed
is ready toTrue
. pygame.KEYUP
: If a secret is launched:- If the launched secret is the spacebar,
space_pressed
is ready toFalse
.
Player Movement:
- The participant’s place (
player_rect
) is up to date based mostly on arrow key enter. - The participant’s x-coordinate is adjusted by the distinction between the precise and left arrow keys multiplied by the participant’s pace.
- The participant’s y-coordinate is adjusted by the distinction between the down and up arrow keys multiplied by the participant’s pace.
- The participant’s place is constrained to remain throughout the display boundaries.
Spawn Targets:
- A counter (
target_spawn_counter
) is incremented in every iteration. - If the counter exceeds the goal spawn fee (
TARGET_SPAWN_RATE
), a brand new goal is created at a random x-coordinate throughout the display width and a hard and fast y-coordinate above the display’s backside edge. - The goal is represented by a rectangle (
target_rect
) added to thetargets
checklist. - The counter is reset.
Update Star Animation:
- The animation of stars is up to date by growing their measurement. If the dimensions exceeds 3, it’s reset to 1.
- The colour of every star is ready based mostly on the present degree.
This a part of the code handles person enter, participant motion, goal spawning, and updates the star animation. It’s an important part of the sport loop, guaranteeing participant interplay and development within the recreation.
How to render the weather of the sport (participant, property, and so forth)
The subsequent a part of the code handles the rendering of recreation parts on the display, together with stars, the grassy space, the participant’s spaceship, and targets. It additionally manages the drawing of the tractor beam and handles collisions between the tractor beam and targets.
# Clear the display
display.fill(BLACK)
# Draw stars with level-based colour
for star in stars:
pygame.draw.circle(display, star['color'], (star['x'], star['y']), int(star['size']))
# Draw the grassy space
pygame.draw.rect(display, GRASS_GREEN, grass_rect)
# Draw the participant and targets
display.blit(ovni, player_rect)
for goal in targets:
display.blit(cow, goal)
# Draw the tractor beam when spacebar is pressed
if space_pressed:
tractor_beam_rect = pygame.Rect(player_rect.centerx - 2, player_rect.centery, 4, HEIGHT - player_rect.centery)
pygame.draw.line(display, YELLOW, (player_rect.centerx, player_rect.centery),
(player_rect.centerx, HEIGHT), 2)
# Check for collisions with targets
for goal in targets[:]:
if tractor_beam_rect.colliderect(goal):
# Change the colour of the tractor beam to yellow
pygame.draw.line(display, YELLOW, (player_rect.centerx, player_rect.centery),
(player_rect.centerx, goal.backside), 2)
# Change the colour of the goal to purple
pygame.draw.rect(display, RED, goal)
targets.take away(goal)
current_score += 1
rating += 1
Code rationalization:
Clear the Screen:
display.fill(BLACK)
: Fills the complete display with a black colour, successfully clearing the earlier body.
Draw Stars:
- Iterates via the checklist of stars (
stars
) and attracts every star as a circle on the display. - The circle’s colour is decided by the star’s colour attribute, and its place and measurement are based mostly on the star’s x and y coordinates and measurement.
Draw Grassy Area:
pygame.draw.rect(display, GRASS_GREEN, grass_rect)
: Draws a rectangle representing the grassy space on the backside of the display. The colour is ready toGRASS_GREEN
.
Draw Player and Targets:
display.blit(ovni, player_rect)
: Draws the participant’s spaceship (ovni
) on the display on the place specified byplayer_rect
.for goal in targets: display.blit(cow, goal)
: Draws every goal (cow) from thetargets
checklist on the display at their respective positions.
Draw Tractor Beam (When Spacebar is Pressed):
- Checks if the spacebar is pressed (
space_pressed
isTrue
). - If true,
tractor_beam_rect
: Creates a rectangle representing the tractor beam based mostly on the participant’s place. pygame.draw.line
: Draws a yellow line representing the tractor beam from the participant’s middle to the underside of the display.- Checks for collisions between the tractor beam and targets.
- If a collision is detected, the goal is eliminated, the tractor beam colour adjustments to yellow, and the goal’s colour adjustments to purple.
- The rating is up to date.
Game logic
Now you might be virtually finished – however first it is advisable to add some logic within the recreation just like the countdown timer which supplies you one minute per degree. You additionally want to attract like a navbar, a easy one with 4 parts containing the overall rating, degree indicator, timer and abductions.
Here’s how we’ll do all that:
info_line_y = 10 # Adjust the vertical place as wanted
info_spacing = 75 # Adjust the spacing as wanted
# Draw the rating in an orange rectangle on the high left
score_text = font.render(f"Score: {rating}", True, WHITE)
score_rect = score_text.get_rect(topleft=(10, info_line_y))
pygame.draw.rect(display, ORANGE, score_rect.inflate(10, 5))
display.blit(score_text, score_rect)
# Draw the extent indicator in a light-blue rectangle on the high left (subsequent to the rating)
level_text = font.render(f"Level: {current_level}", True, WHITE)
level_rect = level_text.get_rect(topleft=(score_rect.topright[0] + info_spacing, info_line_y))
pygame.draw.rect(display, LIGHT_BLUE, level_rect.inflate(10, 5))
display.blit(level_text, level_rect)
# Draw the countdown timer in a purple rectangle on the high left (subsequent to the extent)
timer_text = font.render(f"Time: {int(countdown_timer)}", True, WHITE)
timer_rect = timer_text.get_rect(topleft=(level_rect.topright[0] + info_spacing, info_line_y))
pygame.draw.rect(display, RED, timer_rect.inflate(10, 5))
display.blit(timer_text, timer_rect)
# Draw the targets to amass for the present degree in a grey rectangle on the high left (subsequent to the timer)
targets_text = font.render(f"Abductions: {current_score}/{abduction_target}", True, WHITE)
targets_rect = targets_text.get_rect(topleft=(timer_rect.topright[0] + info_spacing, info_line_y))
pygame.draw.rect(display, GRAY, targets_rect.inflate(10, 5))
display.blit(targets_text, targets_rect)
# Update the show
pygame.show.flip()
# Cap the body fee
clock.tick(FPS)
Code rationalization:
Info Line Positioning:
info_line_y = 10
: Specifies the vertical place for the data line on the high of the display.info_spacing = 75
: Sets the spacing between completely different items of data on the road.
Draw Score:
score_text
: Renders the rating textual content utilizing the sport font.score_rect
: Retrieves the rectangle that encloses the rendered rating textual content.pygame.draw.rect
: Draws an orange rectangle across the rating textual content, making a background.display.blit(score_text, score_rect)
: Blits (renders) the rating textual content onto the display.
Draw Level Indicator:
level_text
: Renders the extent indicator textual content.level_rect
: Retrieves the rectangle for the extent indicator textual content.pygame.draw.rect
: Draws a light-blue rectangle across the degree indicator textual content.display.blit(level_text, level_rect)
: Blits the extent indicator textual content onto the display.
Draw Countdown Timer:
timer_text
: Renders the countdown timer textual content.timer_rect
: Retrieves the rectangle for the countdown timer textual content.pygame.draw.rect
: Draws a purple rectangle across the countdown timer textual content.display.blit(timer_text, timer_rect)
: Blits the countdown timer textual content onto the display.
Draw Abduction Targets:
targets_text
: Renders the textual content indicating the variety of abductions required for the present degree.targets_rect
: Retrieves the rectangle for the kidnapping targets textual content.pygame.draw.rect
: Draws a grey rectangle across the abduction targets textual content.display.blit(targets_text, targets_rect)
: Blits the kidnapping targets textual content onto the display.
Update Display:
pygame.show.flip()
: Updates the show to mirror the adjustments made on this iteration.
Frame Rate Cap:
clock.tick(FPS)
: Caps the body fee to the required frames per second (FPS
). This ensures that the sport runs at a constant pace throughout completely different techniques.
And now we’re prepared for the final code block. It comprises the countdown timer logic that is associated to the extent development logic and the abductions reset. This implies that it is advisable to restart the abductions made by the participant to zero each time you degree up, for instance:
- Level 1: 0/10 abductions
- Level 2: 0/20 abductions
And so on – the utmost abductions quota is 100 abductions in degree 10, including 10 abductions to the required quota for each degree.
Timer logic and quitting perform
Check out the final block of code:
# Countdown Timer Logic
countdown_timer -= 1 / FPS # Decrease the timer based mostly on the body fee
if countdown_timer <= 0:
# Check if the participant reached the kidnapping goal for the present degree
if current_score < abduction_target:
# Player did not attain the kidnapping goal, finish the sport
game_over_screen(display)
working = False
else:
# Move to the subsequent degree
current_level += 1
if current_level <= 10:
current_score = 0
abduction_target = 10 * current_level
countdown_timer = 60 # Reset the countdown timer for the subsequent degree
# Reset the targets textual content for the subsequent degree
targets_text = font.render(f"Abductions: {current_score}/{abduction_target}", True, WHITE)
targets_rect = targets_text.get_rect(topleft=(timer_rect.topright[0] + info_spacing, info_line_y))
# Check if the participant reached the kidnapping goal for the present degree
if current_score >= abduction_target:
# Move to the subsequent degree
current_level += 1
if current_level <= 10:
current_score = 0
abduction_target = 10 * current_level
countdown_timer = 60 # Reset the countdown timer for the subsequent degree
# Reset the targets textual content for the subsequent degree
targets_text = font.render(f"Abductions: {current_score}/{abduction_target}", True, WHITE)
targets_rect = targets_text.get_rect(topleft=(timer_rect.topright[0] + info_spacing, info_line_y))
else:
victory_screen(display)
working = False
# Quit Pygame
pygame.stop()
Countdown Timer Logic:
countdown_timer -= 1 / FPS
: Decreases the countdown timer by the fraction1 / FPS
. This adjustment ensures that the timer decreases uniformly based mostly on the body fee.
Check Timer Expiration:
if countdown_timer <= 0:
: Checks if the countdown timer has reached or fallen beneath zero.
Player Didn’t Reach Abduction Target:
if current_score < abduction_target:
: Checks if the participant’s present rating is lower than the kidnapping goal for the present degree.game_over_screen(display)
: Calls the perform to show the sport over display.working = False
: Sets theworking
flag toFalse
, terminating the sport loop.
Move to Next Level:
else:
: Executes when the participant has reached the kidnapping goal for the present degree.current_level += 1
: Increments the present degree.if current_level <= 10:
: Checks if there are extra ranges to proceed to.current_score = 0
: Resets the present rating for the subsequent degree.abduction_target = 10 * current_level
: Sets the kidnapping goal for the subsequent degree.countdown_timer = 60
: Resets the countdown timer for the subsequent degree.- Resets the targets textual content for the subsequent degree.
targets_text = font.render(f"Abductions: {current_score}/{abduction_target}", True, WHITE)
targets_rect = targets_text.get_rect(topleft=(timer_rect.topright[0] + info_spacing, info_line_y))
Player Reached Abduction Target for All Levels:
else:
: Executes when the participant has efficiently accomplished all ranges (reached degree 10).victory_screen(display)
: Calls the perform to show the victory display.working = False
: Sets theworking
flag toFalse
, terminating the sport loop.
Quit Pygame:
pygame.stop()
: Cleans up and quits Pygame after the sport loop has ended.
Now, you are finished and you may run the sport by typing this in your shell:
python aliend_aductions_game.py
If every thing it is okay, it’s best to see the beginning display that can allow you to play after you press any key.
Conclusion
We’ve lined rather a lot on this tutorial. You began out by establishing the event atmosphere, putting in PyGame, and designing the sport idea with easy mechanics.
Then you adopted the step-by-step means of coding the sport, from initializing the atmosphere to implementing participant controls, collisions, and dynamic parts. You discovered the right way to combine photographs for the spaceship and cows, creating a fascinating visible expertise.
The recreation options varied ranges, every with its personal problem and a countdown timer, including a component of urgency. You applied a scoring system, degree development, and interesting graphics to boost the participant expertise.
Encouragement for additional studying:
Congratulations on reaching this level! Game improvement is a dynamic and rewarding studying path, and there is all the time extra to be taught. As you proceed, think about delving into extra superior subjects akin to:
- Advanced graphics: improve your recreation with extra detailed graphics, animations, and visible results.
- Sound and music: combine sound results and background music to raise the gaming expertise.
- Game physics: discover real looking actions and interactions throughout the recreation world.
- Multiplayer performance: discover ways to implement multiplayer options for a extra interactive expertise.
- Optimization methods: dive into optimizing your code and graphics for higher efficiency.
If you attain this level, thanks for studying and I hope you take pleasure in this submit as a lot as I loved doing it, additionally you may examine the unique code of this recreation and obtain the property from right here:
GitHub – jpromanonet/alien_abduction_game: A humorous alien abduction recreation written in python with pygame.
A humorous alien abduction recreation written in python with pygame. – GitHub – jpromanonet/alien_abduction_game: A humorous alien abduction recreation written in python with pygame.