If you’ve hung out on HackerRank or LeetCode, you might need seen many of the optimum options use Set Theory. By studying this text, you’ll acquire a deeper data into set idea and use it to create optimum algorithms.
Set Theory was invented (or discovered, relying on the way you view maths) by George Cantor.
It revolves across the thought of units, as in collections of objects. Set idea is extremely highly effective and can be utilized to put in writing some lovely & elegant code.
🤔 What is a Set?
A set is just a set of objects. They don’t should be the identical sort or have any relation to 1 one other.
set = (apples, pears, oranges)
Sets are denoted with parenthesis ( )
. With the separation of components being a comma. Or typically { }
. Sets comply with 2 guidelines:
Sets can solely comprise distinctive objects. It can’t comprise the identical merchandise twice or extra.
There is not any order or construction to a set. The first rule is kind of helpful. Say, for instance, now we have a listing of phrases from a guide:
phrases = ["hello", "my", "name", "is", "brandon", "and",
"your", "name", "is", "brandon"]
To discover all of the distinctive phrases, we put the phrases right into a set:
phrases = ["hello", "my", "name", "is", "brandon", "and",
"your", "name", "is", "brandon"]
distinctive = set(phrases)
# {'howdy', 'is', 'and', 'brandon', 'my', 'your', 'identify'}
The 2nd rule ties in properly with the first rule. A set goals to copy how we see issues in our lives.
Imagine we’re on a highway journey with Jahan, Olivia, and Ryan.
Next 12 months, we need to go on a subject journey once more. It doesn’t make a lot sense to ask Jahan, Olivia, Jahan, Ryan, and Jahan.
And it additionally doesn’t make sense to assign an “order” to them. They’re folks, not components in an array! There are different sorts of units too. Such as a multiset which permits non-unique components however no order.
🦁 Cardinality
The cardinality of a set is the size of the set. For the set:
$$A = {1, 2, 3, 4, 5, 6}$$
The cardinality is:
$$|A| = 6$$
🚴♀️ Equality of Sets
2 units are equal when all the weather match.
$$a = {1, 2, 3}$$
$$b = {3, 2, 1}$$
$$a == b$$
Remember, the order doesn’t matter – so units are equal regardless of being within the ‘mistaken’ order!
🚇 Infinite Sets
Some units are infinite. Such because the set containing all of the pure numbers, or all the actual numbers.
We can specific infinite units utilizing a bit of little bit of maths. Heard of record comprehensions? It seems to be the identical syntactically however operates infinitely.
The set of all even numbers is:
$$A = {2n: n in mathbb{Z}}$$
Read this as “For every quantity, n, within the set of all integers, Z, multiply the quantity by 2”. Which will give us the set of all even numbers.
Which will give us the set of all even numbers.
In a listing comprehension, it might seem like this:
[2 * n for n in naturalNumbers]
However, this gained’t work as Python doesn’t enable infinite units.
🐈⬛ The Empty Set
The empty set is the set containing nothing in any respect. It is very similar to 0. It is nothing and serves the aim of a negated worth. We don’t have 0 apples, now we have no apples.
The empty set serves the identical objective. We don’t have a set containing all of the phrases, now we have nothing.
Its image is ∅
🌌 The Universal Set
The Universal Set, U, is the set which comprises all objects, together with itself. If our universe comprises solely integers, 1, 2, 3, …, then the common set is the set of all integers.
🏥 Operations
Just like with different knowledge varieties, units have operations! Let’s begin with some operations it’s possible you’ll already know.
❌ Union
The union is combining 2 units collectively (suppose addition). The image for the union is ∪. Let’s take a look at an instance.
$$A = {1, 2, 3}$$
$$B = {3, 4, 5}$$
$$B cup A = {1, 2, 3, 4, 5}$$
Notice how after we carry out a union, now we have two threes. Since units solely comprise distinctive components, we merely toss the additional 3 away. Also word, there is no such thing as a order. So B ∪ A doesn’t lead to (3, 4, 5…) because the order doesn’t exist.
We may even say B ∪ A = {3, 5, 1, 4, 2}. What if we union an empty set with a non-empty set? Or an empty set with one other empty set?
$$varnothing cup {1, 2, 3} = {1, 2, 3}$$
$$varnothing cup varnothing = varnothing$$
When we get onto subsets and the like we’ll be taught to understand the empty set and this simplistic maths.
🦄 Intersection
The intersection of a set is each merchandise in set A that can also be in set B.
Think of it just like the boolean operator AND. Its image is ∩.
$$A = {1, 2, 3}$$
$$B = {3, 4, 5}$$
$$A cap B = {3}$$
Let’s say now we have a listing of flights with passengers on board. And now we have a listing of the people who took my daughter (Taken reference).
We can use the intersection operation to search out the record of flights that comprise the individuals who took my daughter.
Because units solely comprise distinctive components, it is vitally quick to calculate this. However, creating the set itself might take time. But, if we have no idea all of the names of the folks within the youngster trafficking ring we are able to construct the set first and use the intersection to question it.
💍 Belongs To
We can create units which belong to different units.11 belongs to set 1,2,31,2,3. In notation, that is:
$$1 in {1, 2, 3}$$
We say that “1 is within the set {1, 2, 3}”.
But don’t get caught out!
$${1} in {1, 2, 3}$$
That shouldn’t be true. We say the component shouldn’t be in ∉ the set.
$${1} notin {1, 2, 3}$$
It could be true if:
$${1} in {{1}, 2, 3}$$
This is a really, very helpful function. Let’s say now we have the phrase “FLAG{” that we need to discover in some textual content. We flip the textual content right into a set, like so:
textual content = "FLAG{"
corpus = set("Hello", "my", "identify", "is")
if textual content in corpus:
print("It's in there!")
Because units don’t comprise distinctive objects, that is slightly quick. However, you need to bear in mind that turning it right into a set is pricey.
🛩️ Subset
authentic = {1, 2, 3, 4}
subset1 = {1}
subset2 = {3, 4}
subset3 = {4, 3, 2, 1}
The image for subset is ({1} subset {1, 2}).
The empty set is a subset of all units, however it’s not a component of all units.
Fun truth, we are able to do that:
$$ mathbb{U} – varnothing$$
This equates to a brand new set, which comprises all the weather of the set aside from the empty set. However, it isn’t the common set – it’s a brand new set!
The energy in subsets, in my view, comes from the energy set.
🔋 Power Set
The Power set is the set of all subsets of a set, together with the empty set and the set itself. For the set {2, 9}
now we have 4 subsets.
{}
{2}
{9}
{2, 9}
The energy set will likely be:
$$frak{P} (mathbb{A}) = emptyset, {2}, {9}, {2, 9}$$
Note: For house use or exams we are able to denote utilizing P(a). For web sites the place we need to impress folks with our fancy use of the alphabet, use LaTeX.
When constructing subsets, the component can both be included within the subset or not. This is binary. It’s both in it, or it isn’t.
That leads us to the system for calculating how giant a powerset is.
$$2^{s}$$
Where s is the dimensions of the set and a pair of is as a result of components are both within the set or not.
Let’s say now we have a listing of substances.
{cabbage, lettuce, tomato, chips, carrots}
It is smart to make use of a set as a result of the order doesn’t matter for a listing of substances and we don’t need to have cabbage twice for some cause.
Now, what number of combos of meals can we make with this?
$$2^{35} = 32$$
The empty set doesn’t rely right here (except we need to starve), so we are able to scale back this to 31.
🥺 Infographic Cheat Sheet for Set Theory
I made this for you! Feel free to print and use it nonetheless you need. Personally, once I was learning for exams, the symbols part proved to be most helpful!
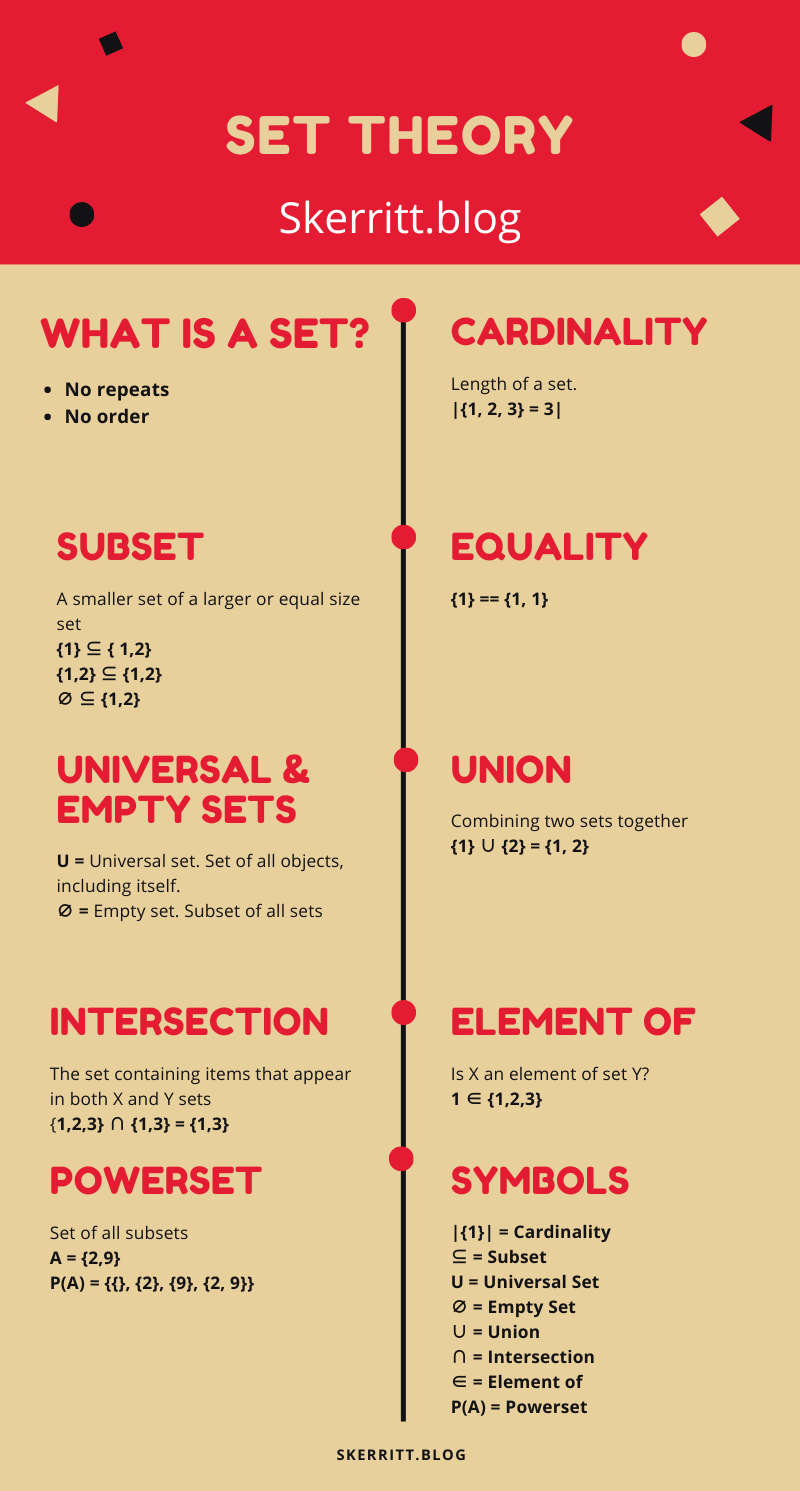
💻 Sets in Programming Languages Are Often Sorted
Not solely do units in most programming languages are sometimes routinely sorted. Take a take a look at this Python code:
>>> x = [9, 8, 7, 6, 5, 4, 3, 2, 1]
>>> set(x)
{1, 2, 3, 4, 5, 6, 7, 8, 9}
And that’s simply the tip of the iceberg. Sets are carried out as hash maps in lots of programming languages. This implies that we get O(1) lookup time. To carry out 6 in x
will take O(1) time. For extra on Big O notation, try my different article.
📖 Dictionary Checking
We have a textual content akin to:
textual content = ["hello", "my", "name", "is", "lkmxjja", "brandon"]
And we need to learn how many phrases in textual content
seem in one other record, text_dict
.
text_dict = ["hello", "hello", "my", "brandon", "name"]
The quickest approach to do that with out utilizing units is to kind each lists after which undergo every one, one by one to rely what number of occasions an merchandise in textual content
seems in text_dict
.
In a real-world instance, we might be checking to see if a textual content is English or not by counting what number of occasions the phrases within the textual content seem within the English dictionary.
By utilizing units, we delete the repetitions in text_dict
. It additionally routinely kinds the 2 units, so it’s a lot simpler for us to look via them. Not to say the O(1) lookup time.
3 birds with 1 stone!
>>> textual content = ["hello", "my", "name", "is", "lkmxjja", "brandon"]
>>> text_dict = ["hello", "hello", "my", "brandon", "name"]
>>> textual content = set(textual content)
>>> text_dict = set(text_dict)
>>> textual content.intersection(text_dict)
{'brandon', 'identify', 'howdy', 'my'}
>>> len(textual content.intersection(text_dict))
4
👋 Conclusion
Set idea is gnarly, and extremely helpful in not simply aggressive programming however every kind of programming. We’ve not solely learnt the speculation behind set idea but in addition how programming languages akin to Python have carried out it.
However, looping via each of them ourselves is unruly. We’d have to put in writing a loop and hold observe of it ourselves. Luckily, we are able to use set theories’ intersection perform to do that for us.